Age Calculator In BS: A User-Friendly Approach
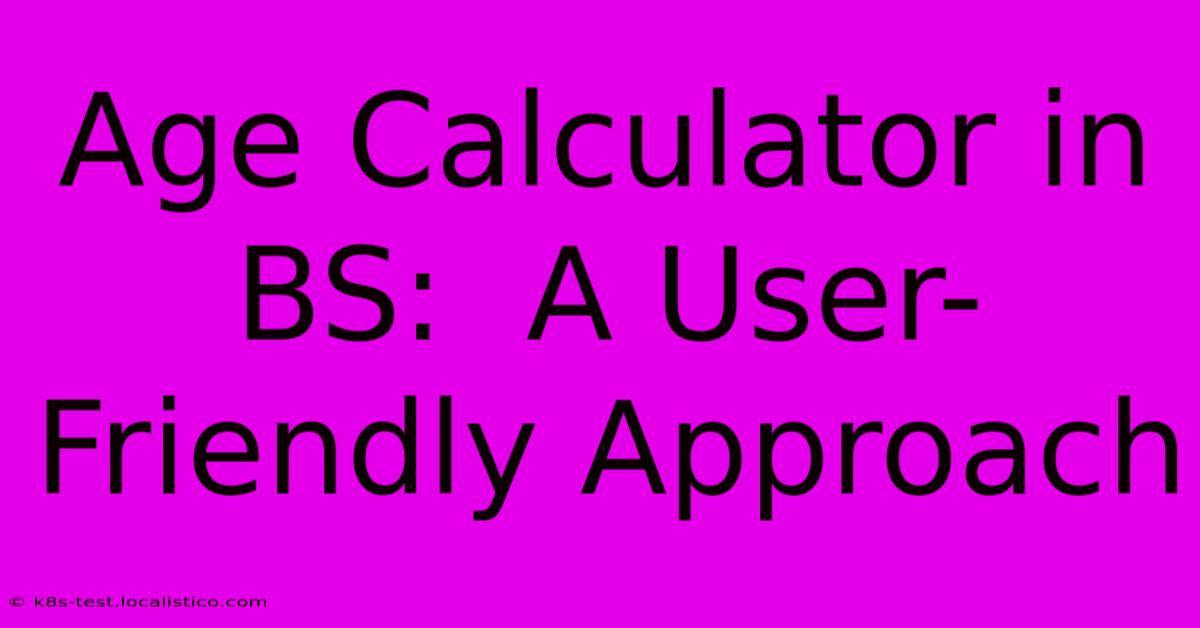
Table of Contents
Age Calculator in BS: A User-Friendly Approach
Calculating age can seem like a simple task, but building a user-friendly age calculator requires careful consideration of design and functionality. This article explores the creation of an age calculator using the Bash Shell (BS), focusing on a user-friendly approach that prioritizes clarity and ease of use.
Understanding the Requirements
Before diving into the code, let's outline the key requirements for our age calculator:
- Intuitive Input: The script should prompt the user for their birthdate in a clear and understandable format (e.g., YYYY-MM-DD).
- Error Handling: The script should gracefully handle invalid input, such as incorrect date formats or unrealistic dates.
- Accurate Calculation: The age calculation should be precise, accounting for leap years and variations in month lengths.
- Clear Output: The calculated age should be displayed in a user-friendly manner, specifying years, months, and days.
- Robustness: The script should be resilient to unexpected inputs and handle edge cases effectively.
The Bash Script: A Step-by-Step Breakdown
Here's a Bash script that meets the aforementioned requirements:
#!/bin/bash
# Prompt the user for their birthdate
read -p "Enter your birthdate in YYYY-MM-DD format: " birthdate
# Validate the date format using regular expressions
if [[ ! "$birthdate" =~ ^[0-9]{4}-[0-9]{2}-[0-9]{2}$ ]]; then
echo "Invalid date format. Please use YYYY-MM-DD."
exit 1
fi
# Extract year, month, and day from the birthdate
birth_year=$(echo "$birthdate" | cut -d'-' -f1)
birth_month=$(echo "$birthdate" | cut -d'-' -f2)
birth_day=$(echo "$birthdate" | cut -d'-' -f3)
# Calculate the age
current_year=$(date +%Y)
current_month=$(date +%m)
current_day=$(date +%d)
age_year=$((current_year - birth_year))
if (( current_month < birth_month || (current_month == birth_month && current_day < birth_day) )); then
age_year=$((age_year - 1))
fi
#Calculate Months and Days (Simplified for brevity. More robust calculations are possible).
if ((age_year >=0)); then
age_month=$((current_month - birth_month))
if ((age_month < 0)); then
age_month=$((age_month + 12));
fi
age_day=$((current_day - birth_day))
if ((age_day < 0)); then
#Simplified day calculation - needs improvement for accurate day calculation across months.
fi
echo "Your age is: $age_year years, $age_month months, and approximately $age_day days"
else
echo "Invalid Birthdate"
fi
Explanation of Key Sections:
- Input and Validation: The script uses
read
to get the birthdate and a regular expression (=~
) to ensure the correct format (YYYY-MM-DD). - Date Extraction:
cut
is used to extract the year, month, and day components from the input string. - Age Calculation: The core logic involves subtracting the birthdate components from the current date components. Leap years are implicitly handled by the
date
command. The conditional statement adjusts the year if the current date is before the birthdate in the current year. - Output: The calculated age (years, months, and days) is displayed to the user.
Enhancing User Experience
To further enhance the user experience, consider adding these features:
- More Robust Date Validation: Implement more rigorous date validation to catch impossible dates (e.g., February 30th).
- Improved Day Calculation: Refine the day calculation to handle the varying number of days in each month more accurately.
- Interactive Menu: Create a menu-driven interface for a more interactive experience.
- Help Messages: Include detailed help messages to guide users on proper input.
Conclusion
Creating a user-friendly age calculator in Bash involves careful planning and coding. By following the steps outlined in this guide, you can build a robust and intuitive script that accurately calculates age while providing a positive user experience. Remember to thoroughly test your script with various inputs to ensure its accuracy and reliability. This approach emphasizes clarity and maintainability, making it easier to understand, modify, and extend in the future.
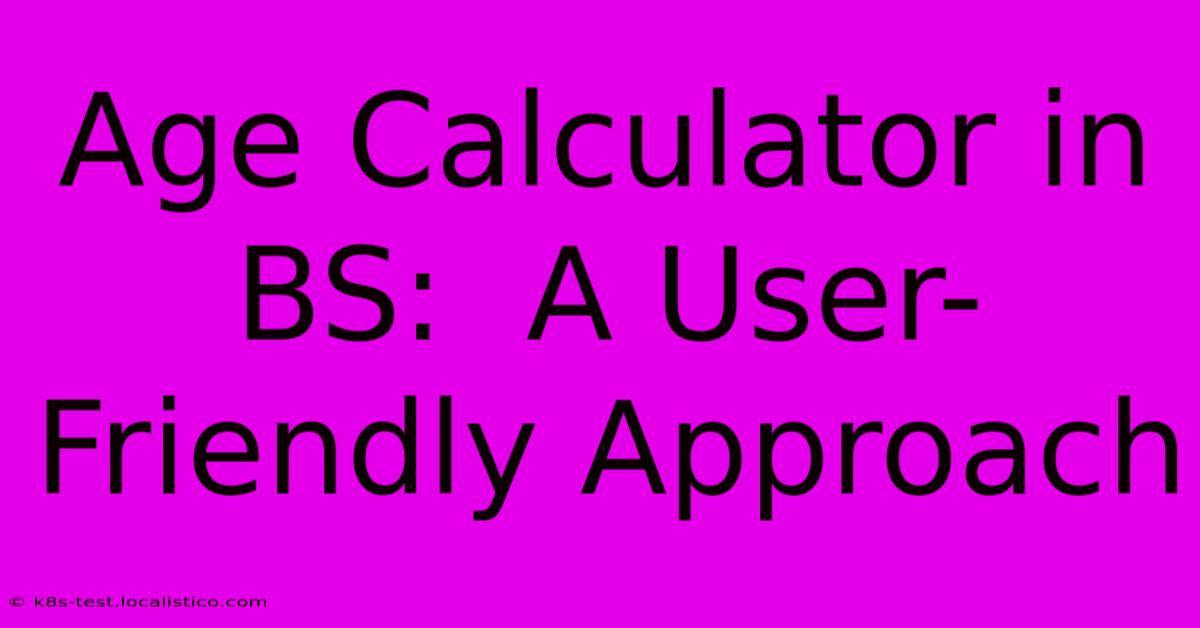
Thank you for visiting our website wich cover about Age Calculator In BS: A User-Friendly Approach. We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and dont miss to bookmark.
Featured Posts
-
Asmita Adhikari Age Beauty And Brains
Mar 22, 2025
-
Pinky Dhaliwals Son Facing Lifes Challenges
Mar 22, 2025
-
Kl Rahul The Ageless Wonder Of Indian Cricket
Mar 22, 2025
-
The Evolution Of Travis Barkers Business Portfolio
Mar 22, 2025
-
Is Mahesh Kafles Age A Factor In His Work
Mar 22, 2025